Week 2: Move/Rotate Mechanic
Hello again! This week I worked on adding a feature to a pre-existing trigger prefab to move and rotate objects when the player enters the collision zone. I definitely learned a lot this week.
Before that, the first change I made was removing the fall speed limit to give the player more momentum:

I started working on this new mechanic with no Unity coding or C# experience, some rusty Java and a dream. In my first pass, I had the idea to change the transform position of the object and change it incrementally using a for loop. This is where I learned what Time.deltaTime was. I copied the use of a list of objects from the Grow objects feature already present in the code (as a reminder, the framework for this code was provided by my professor Michael Baker).
Then, in the block that executes when the player is inside the trigger's collision zone, I added the for loops manually, checking that the list is not empty and the direction that the object would move in:
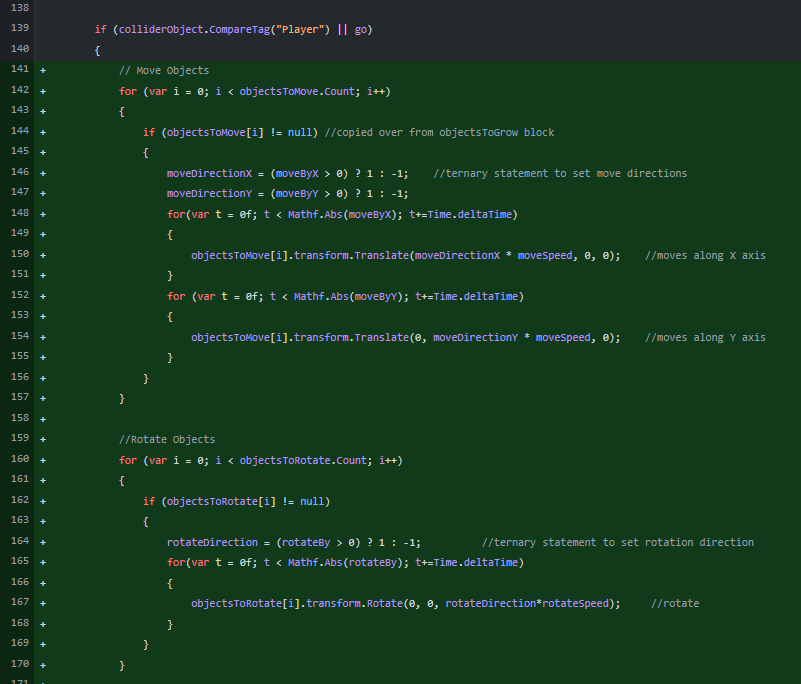
This... didn't work well. Once I fixed my compile errors, the object would move immediately without any incremental motion. It also moved in unintended units. For example I would input -1.1 into my rotateBy variable (which should be in degrees) but it would appear to rotate -80 degrees.
Little did I know incremental movement can be done super easily using Lerp and SmoothDamp. My professor also gave me tips on how to organize in scripts. I moved the new code into the pre-existing ResizeObject script and renamed it to TransformObject, to include the new move/rotate mechanic.
I used Quaternion.Slerp and Vector3.SmoothDamp to create smooth incremental movement after experiencing issues with Lerp, which uses linear interpolation. Here are a few of the fails:
Here I was using Lerp for movement on the blue platforms (which are kinematic objects), but realized that the update method to change the transform that I was using also affects dynamic objects which have the TransformObject script attached. They jittered so much since the physics engine and the update method were trying to affect the object at the same time.
Before I realized this, I was tweaking variables and accidentally caused a crash. I of course expressed my frustration in the best way possible: posting about it.
To fix this issue, I used GetComponent to get the Rigidbody2D component from the object that the TransformObject script is attached to, to only affect kinematic boxes. I also added a check to not affect objects that do not have a Rigidbody2D component.
Here's my finished code from TransformObject:
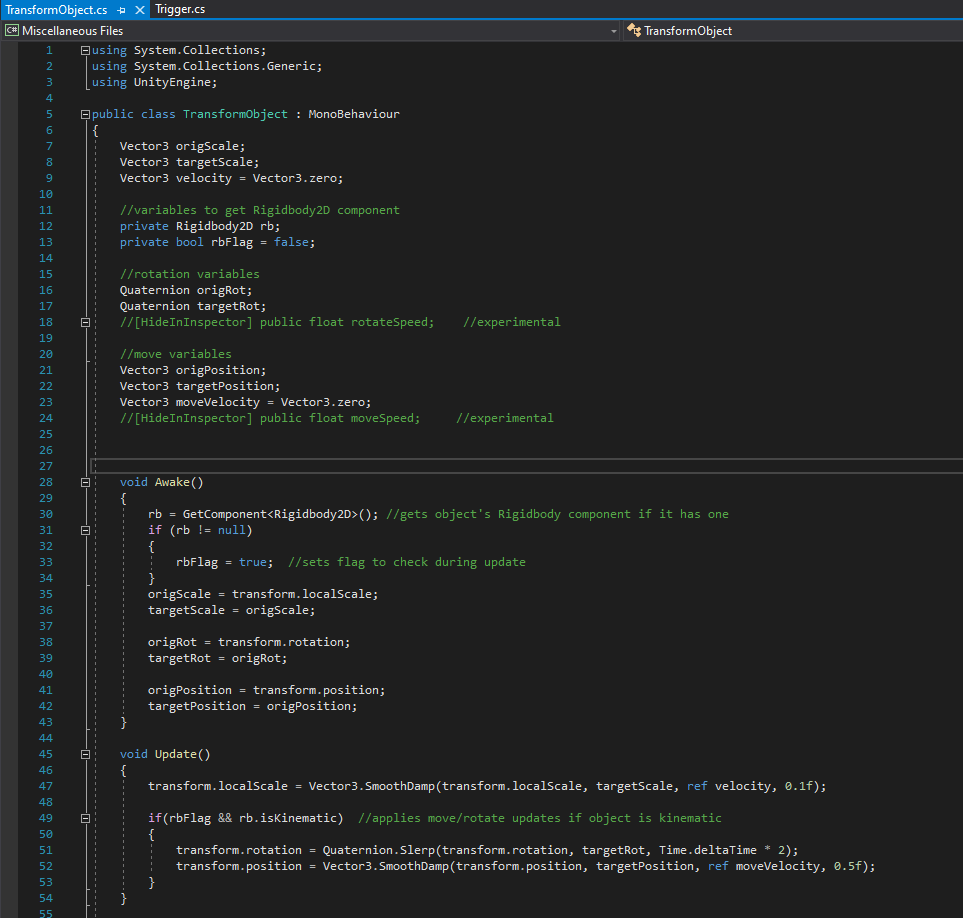
After adding code into TransformObject, I cleaned up the Trigger script a bit, and here's the result:
Above are variable declarations. I was planning on implementing speed, but found the static speed set in TransformObject to work well. Below are the calls within the trigger collision block:
They now reference methods in TransformObject instead of being directly in the script, making it easier to read.
And here's a test using Slerp and SmoothDamp for motion:
With that, the move/rotate mechanic now works!
Finally, I also did more work on level art, starting my tileset and making a mockup to see how different elements mesh and tile together. Here's the tileset so far:
And here's the mockup:
I've been enjoying making small changes to copies of some tiles to add more variety, like making cracks in the bricks and notches onto pipes. I'd like to add perhaps a bit more contrast in the foreground, but this is just the first version.
I also learned a lot about Unity this week, and have gotten more familiar with its methods and interactions between scripts. Until next week!
ECK-423
Level 2 of ECK-423 (Discontinued for now)
Status | In development |
Author | Boltcry |
More posts
- Week 12: Revising Level 2Apr 07, 2023
- Weeks 9-11: Level 2 Design (Part 2)Mar 29, 2023
- Week 8: Level 2 Design (Pt 1)Mar 08, 2023
- Week 7: Serialized Transform ClassFeb 28, 2023
- Week 6: Checkpoint SystemFeb 21, 2023
- Week 5: Play DiscoveriesFeb 14, 2023
- Week 4: Move/Rotate Timer & GUI FeaturesFeb 07, 2023
- Week 3: Code optimizationJan 31, 2023
- Week 1: Organization & Controller UpdatesJan 17, 2023
Leave a comment
Log in with itch.io to leave a comment.